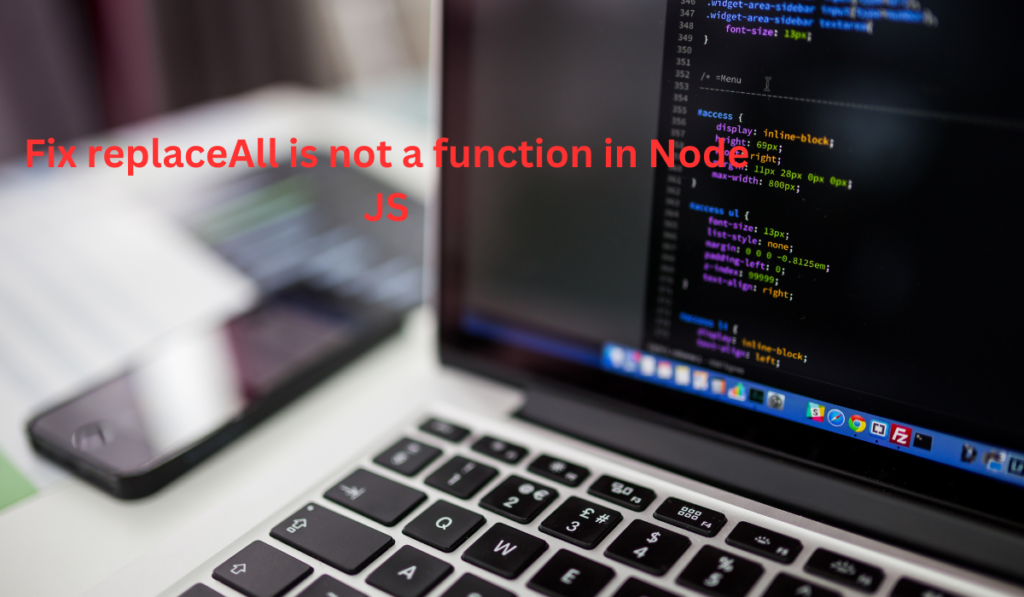
If you’re encountering the “replaceAll” is not a function error in NodeJS, you can easily fix it with the following solutions.
The replace function is a commonly used string prototype in JavaScript, allowing you to replace the first instance of a specific substring with any desired string.
However, if you need to replace all occurrences of that substring, you might consider using replaceAll function in the NodeJS environment. However, this function may not work as expected. Let’s examine an example to understand what’s happening.
let input = “00aa00bb00cc00dd”
let output = input.replaceAll(’00’, ”);
console.log(output)
//OUTPUT
//TypeError: input.replaceAll is not a function
The above code functions perfectly on all major browsers, except for Internet Explorer. However, if you attempt to utilize it within a NodeJS version 14 or lower environment, an error message will appear stating that replaceAll is not a function.
Why does this occur? In NodeJS versions prior to 15, “replaceAll” is not a native string prototype, resulting in an error. The following alternatives may be used instead.
How to replaceAll in Node JS
If you’re receiving an error message while using replaceAll in Node JS, it’s because this method is only available in Node versions 15 and newer.
Upgrading your Node environment can resolve this issue, but you can also use the standard “replace” function with a regular expression as an alternative to replaceAll. In the following steps, we will explain how to use this method and how to create a custom replaceAll function for repeated use in Node JS.
Solution 1 – Use replace with regex
Instead of just replacing the first occurrence of a string within another string, we can use a regular expression to match and replace all instances of the string. Here’s an example of how to do it using the replace function in JavaScript:
let input = “00aa00bb00cc00dd”
let output = input.replace(/00/g,”)
console.log(output)
//OUTPUT
//aabbccdd
The regular expression we’re using to target our string is enclosed between two forward slashes, and the “g” flag is added to indicate that all occurrences of the string should be replaced. Without the “g” flag, the regular expression would only replace the first occurrence of the string.
Solution 2 – Create your own replaceAll prototype
Below is an example of how to add a custom replaceAll method to the String prototype in JavaScript, enabling you to use the function on any string in your code:
String.prototype.replaceAll = function(search, replace) {
return this.split(search).join(replace);
}
With this function added to the String prototype, you can call replaceAll on any string, like this:
const myString = “Hello world, hello universe!”;
const newString = myString.replaceAll(“hello”, “hi”);
console.log(newString); // Output: “Hi world, hi universe!”
This will replace all occurrences of “hello” in the string with “hi”.
Solution 3 – Update to Node 15+
If you’re facing issues with the replaceAll function in NodeJS, upgrading your Node version to 15 or higher is the easiest solution. However, this may cause issues in other parts of your code.
To avoid such problems, it’s recommended to use a version manager like NVM to install Node. This way, you can easily install the latest version of Node and switch back to an older version if needed. It also helps you to match your production environment with your development environment.