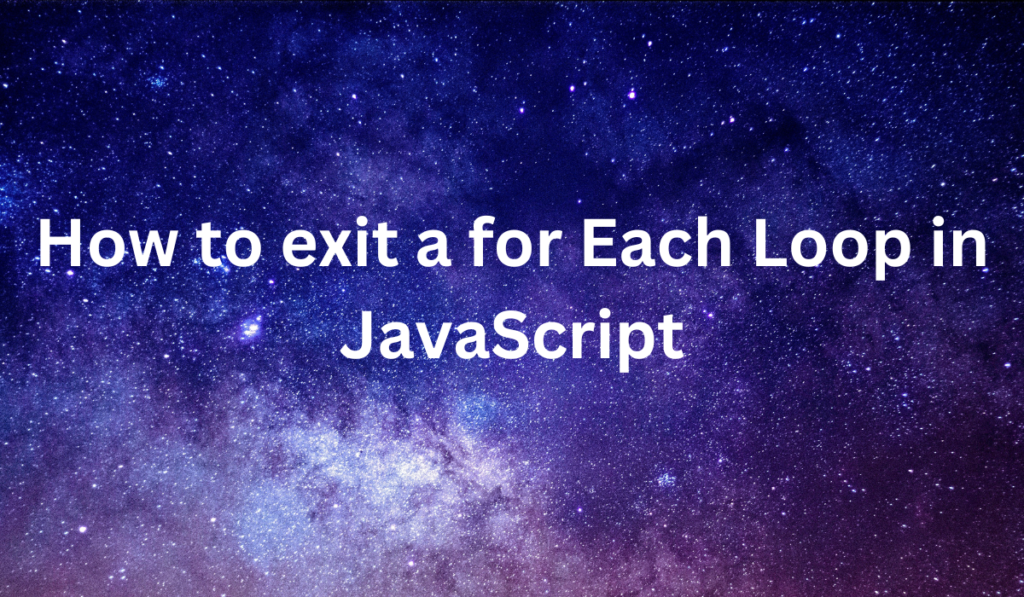
Dealing with loops is an integral part of coding. There are several types of loops in JavaScript, each with its own syntax. It can be overwhelming to make sense of them all, but knowing the appropriate syntax is crucial to becoming a proficient programmer, particularly when faced with multiple options.
One such loop is the forEach loop in JavaScript, which is utilized for iterating over arrays. Despite its simple syntax, complications arise when attempting to perform more complex tasks, like breaking out of the loop. In this article, we will explore how to exit a forEach loop in JavaScript, using the “break” keyword.
How to Exit a for Each Loop in JavaScript
Breaking out of a forEach loop in JavaScript does not have an official or proper way. Attempting to use the typical “break” syntax will result in an error being thrown. In case breaking out of the loop is essential, it is preferable to use a traditional loop instead.
Consider the following code snippet: We expect the code to stop running after finding the name “Steph.” However, it throws an “Unsyntactic break” error.
let data = [{name: ‘Rick’}, {name: ‘Steph’},{name: ‘Bob’] data.forEach(obj => {console.log(obj.name)
if (obj.name === ‘Steph’) { break;}})
Solution
As with many programming challenges, there is a workaround for breaking out of a forEach loop in JavaScript. We can achieve this by throwing and catching an exception while iterating through our array. Here’s an example of how we can accomplish this:
We wrap our forEach loop inside a try-catch block. Instead of using “break” to exit the loop, we use “throw ‘Break'”. We then move down to the catch block and check the error. If it is anything other than “Break”, we throw it up the error chain. Otherwise, we continue executing our code.
While this solution is effective, it is not very elegant. There are better alternatives that we can explore.
let data = [ {name: ‘Rick’},{name: ‘Steph’},{name: ‘Bob’}] try { data.forEach(obj => {console.log(obj.name)
if (obj.name === ‘Steph’) {throw ‘Break’; })} catch (e) { if (e !== ‘Break’) throw e}
Alternative #1: for…of loop (Preferred)
If we want to break out of a loop in JavaScript, for…of loop is a better option compared to forEach loop. It has clean and easy-to-read syntax and allows us to use “break” once again. Additionally, using the for…of loop enables us to perform await operations within it, which is not possible with forEach loop.
let data = [ {name: ‘Rick’},{name: ‘Steph’},{name: ‘Bob’}]
for (let obj of data) {console.log(obj.name) if (obj.name === ‘Steph’) break;}
Alternative #2: every
Another approach to breaking out of a loop in JavaScript is to use the “every” array prototype. Here’s an example code snippet:
The “every” prototype tests each element of the array against our function and expects a Boolean return. When a value is returned as “false”, the loop breaks. In this case, we invert our name test and return “false” when the name is equal to “Steph”. This will break the loop and allow us to continue executing our code.
let data = [{name: ‘Rick’},{name: ‘Steph’},{name: ‘Bob’}]
data.every(obj => {console.log(obj.name) return !(obj.name === ‘Steph’)})
Alternative #3: some
Exiting a forEach loop in JavaScript can be achieved using various alternatives, including the for…of loop, every and some array prototypes, and the throw and catch workaround.
Among the alternatives, for…of loop is preferred due to its clean and readable syntax and the ability to use the break keyword. Additionally, it allows await operations within it, which is not possible with the forEach loop.
Alternatively, every and some array prototypes can also be used to test each element against a function and break the loop when a certain condition is met. Every prototype expects a false return value to break the loop, while some prototypes break the loop with a true return value. Choosing between the two depends on your code’s readability and use case.
In conclusion, while there is no direct way to break out of a forEach loop in JavaScript, you have several options to choose from, each with its own advantages. Remember to consider code readability when selecting the best solution for your code. Happy coding!
let data = [ {name: ‘Rick’},{name: ‘Steph’},{name: ‘Bob’} ]
data.some(obj => { console.log(obj.name) return (obj.name === ‘Steph’)})